Color Mapping
With OpenCV we can use different colorspaces other than RGB, this is the case of HSL and HSV, that stand for Hue-saturation-lightness and hue-saturation-value.
Here how HSL will look like
and here how HSV will look like
Now in in openCV we can transform or convert the different pictures to a different colorspace.
Import Libraries¶
First the imports
now with the imports we read or get the image we are going to use to work with
Converting to Different Colorspaces¶
now, we did this before, we convert from BGR to RGB
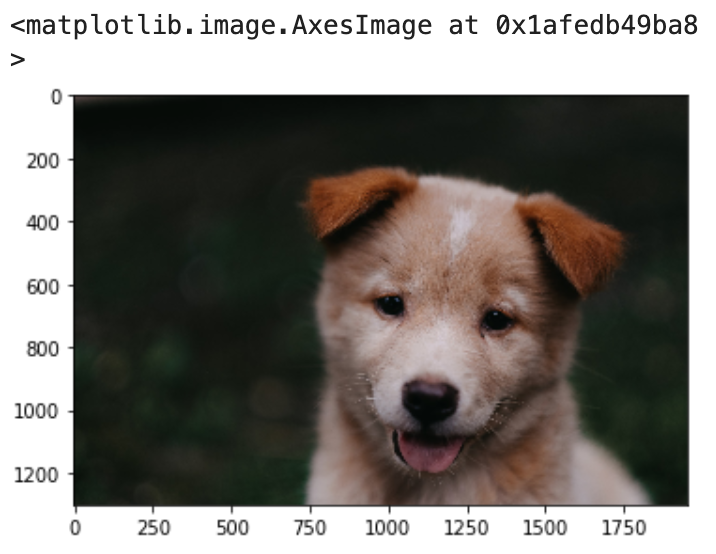
Convert to HSV¶
now, first HSV
Convert to HSL¶
the next one will be HSL